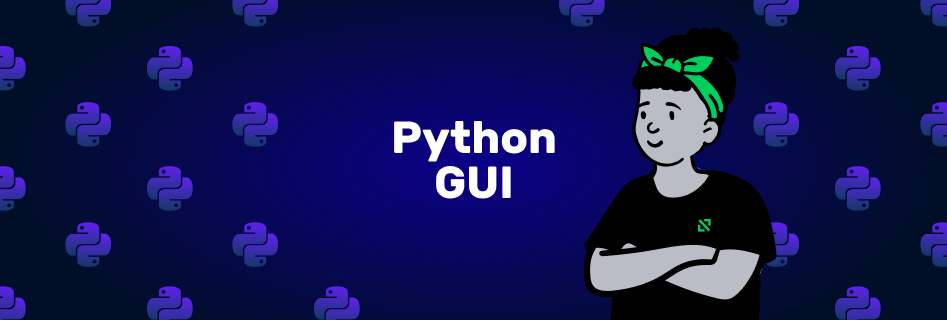
What Is Python GUI Programming
Each coding language you use has its rules and requirements. That is what we use in Python too. That code has several unique features including its well-implemented GUI programming part. To understand the whole essence of GUI you may need to imagine a website and its design. That is what GUI is for a user – a constructive and comprehensible user interface design.
When you start learning Python, you maybe do not actually worry about what is a GUI design. It seems more important to get through the syntax of that coding language, not to design.
What Is Python GUI Coding and What Do You Need for It
Let’s start from the very beginning and comprehend what is Python GUI programming and what is GUI design as a whole. GUI aka Graphical User Interface or a graphical user interface is a form of user interface that allows users to interact with the device using graphical elements (icons, cursors, buttons, etc). Actions in a graphical interface are usually performed by directly manipulating graphical elements.
A graphical interface is considered more convenient than a text-based command-line interface because the user has random access to visible objects using input devices. At the same time, interface elements display properties, which greatly facilitate the user’s work.
For Python graphic interface development, you may need to get through the Python GUI development guide which is not an easy thing to comprehend. There are lots of guides to help you with your GUI Python task yet you should get the knowledge of the basics to use it further.
For a good technology for your Python GUI development, it is great to get the following features:
- Rendering on the GPU.
- Simple built-in support for asynchronous functions.
- Full control of themes and styles.
- Simple built-in logging window.
- Lots of widgets, hundreds of their combinations.
- Detailed documentation, examples and unparalleled support.
With each technology, you choose for making a GUI on Python, these features are crucial.
As usual, there are several interfaces or technologies used for GUI interface design and development. Let’s go through them. We have chosen the best 5 libraries you can use for your Python GUI development.
1.PyQt5
PyQt5 is a graphical user interface (GUI) for Python. It is very popular among developers and GUI can be created by coding or QT designer. The QT development framework is a visual framework that allows you to drag and drop widgets to create user interfaces.
It is a free and open-source binding software and implemented for a cross-platform application development platform. It is used on Windows, Mac, Android, Linux, and Raspberry Pi.
To install PyQt5 you can use the following command:
pip install pyqt5
A simple code is shown here:
from PyQt5.QtWidgets import QApplication, QMainWindow
import sys
classWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setGeometry(300, 300, 600, 400)
self.setWindowTitle(“PyQt5 window”)
self.show()
app = QApplication(sys.argv)
window = Window()
sys.exit(app.exec_())
The ScienceSoft Python development team highlights the benefits of using PyQt:
PyQt is a mature set of Python to Qt bindings for cross-platform desktop application development. It offers a rich selection of built-in widgets and tools for creating custom widgets for complex graphical interfaces, as well as robust SQL database support for connecting to and interacting with databases.
2. Python Tkinter
Another graphics framework is called Tkinter. Tkinter is one of the most popular Python GUI libraries for desktop application development. It’s a combination of the standard TK GUI and python.
Tkinter provides various widgets such as labels, buttons, text boxes, and checkboxes that are used in a GUI application.
Button control widgets are used for displaying and designing applications while canvas widget is used for drawing shapes like lines, polygons, rectangles, etc. in an application. Also, Tkinter is a built-in library for Python, so you don’t need to install it like other graphics frameworks. Below is an example of coding using Tkinter:
from tkinter import *
class Root(Tk):
def __init__(self):
super(Root,self).__init__()
self.title(“Python Tkinter”)
self.minsize(500,400)
root = Root()
root.mainloop()
3.PySide2
The third Python GUI library we’ll be talking about is PySide2, or you can call it QT for python. Qt for Python offers official Python bindings for Qt (PySide2) to allow its API to be used in Python applications and a binding generator tool (Shiboken2) that can be used to represent C++ projects in Python.
Qt for Python is available under the LGPLv3/GPLv3 license and the Qt commercial license.
4. Kiwi
Another graphics framework that we will be talking about is called Kiwi. Kiwi is an open-source Python library for rapidly developing applications that use innovative user interfaces such as multi-touch applications.
Kiwi runs on Linux, Windows, OS X, Android, iOS, and Raspberry Pi. You can run the same code on all supported platforms. It can natively use most inputs, protocols and devices including WM_Touch, WM_Penn, Mac OS X Trackpad and Magic Mouse, Netdev, and Linux HID kernel.
Kiwi is 100% free to use under the MIT license.
The toolkit is professionally designed, maintained and used. You can use it in a commercial product. The framework is stable and has a well-documented API as well as a programming guide to help you get started.
The Kiwi graphics engine is built on OpenGL ES 2 using a modern and fast graphics pipeline.
The toolkit comes with over 20 widgets, all of which are highly extensible. Many parts are written in C using Cython and tested with regression tests.
Before you install Kiwi, you need to install the “glew” dependency. You can use the pip command like below:
pip install docutils pygments pypiwin32 kivy.deps.sdl2 kivy.deps.glew
Type this command and press enter, it will be installed. After that, you need to enter this command to install Kiwi:
pip install Kiwi
So after installation, let me show you a simple Kiwi example to show how easy it is:
from kiwi.app import App
from kiwi.uix.button import Button
class TestApp(App):
def build(self):
return Button(text= ” Hello Kiwi World “)
TestApp().run()
5.wxPython
So, the last graphics framework we’re going to talk about is wxPython. wxPython is a cross-platform graphical toolkit for the Python programming language.
This allows Python programmers to create programs with a robust, highly functional graphical user interface, simply and easily. It is implemented as a set of Python extension modules that wrap GUI components of the popular wxWidgets cross-platform C++ library.
Like Python and wxWidgets, wxPython is open source.
wxPython is a cross platform toolkit. This means that the same program will run on multiple platforms without modification. The following platforms are currently supported: Microsoft Windows, Mac OS X and macOS, and Linux.
Now I will show you the installation process and create a simple example. So to install, just type the following command:
Where to Get Python GUI Tutorial and Assignment Done?
There are several ways on how to make GUI in Python. You can use your own skills creating something new that will be the best option ever for you. Besides, you can delegate the task to the pros from our company, whose help with programming has saved tons of future genius’ names. Their help with Python homework will save your time and help you to cope with Python learning routines easily.